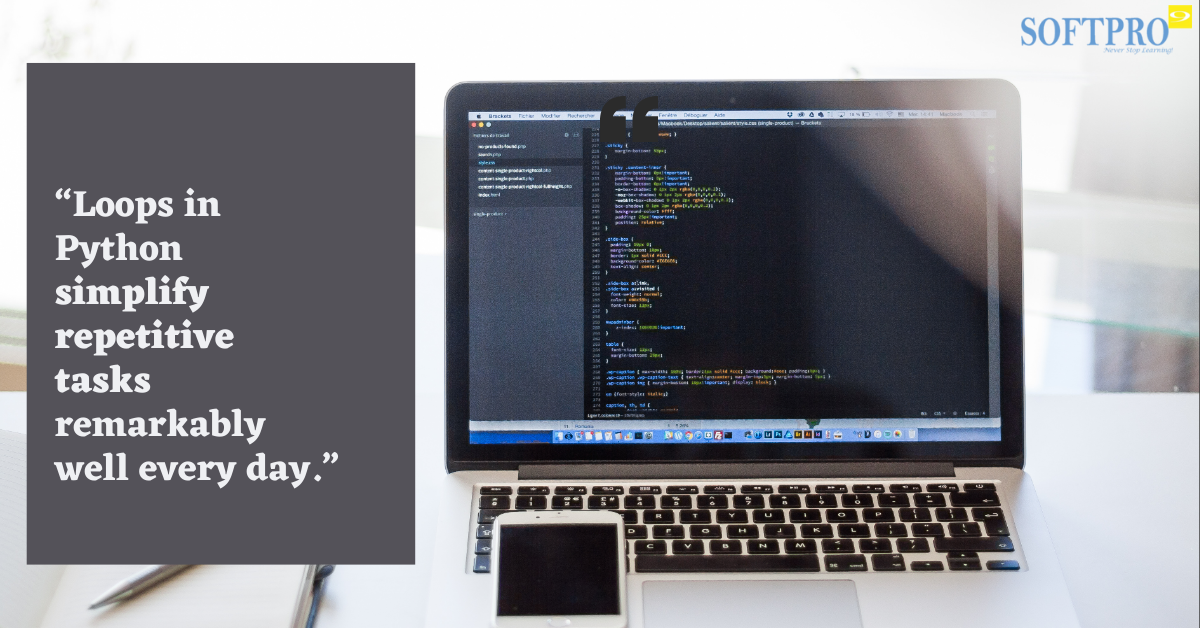
How Many Types of Loops Are Used in Python
#1 Myinstitutes.com is one of the Best Educational Portal and Training Institutes in MYSORE, MANGALORE, and BANGALORE.
Loops are a fundamental concept in Python and all programming languages, enabling developers to execute a block of code repeatedly without rewriting it multiple times. This repetition, or iteration, is a crucial aspect of solving complex problems and automating processes efficiently. Python supports two primary types of loops: for
loops and while
loops. Let’s dive deep into these types, their functionalities, and how to use them.
1. For Loop: Iterating Over a Sequence
The for
loop in Python is primarily used to iterate over a sequence such as a list, tuple, string, set, or dictionary. It is highly intuitive and straightforward, making it a favourite for programmers.
Syntax:
pythonCopy codefor item in sequence:
# Execute this block of code
Use Case:
- When the number of iterations is fixed or predetermined.
- Ideal for iterating through elements in data structures or generating a series of numbers.
Example:
pythonCopy codenumbers = [1, 2, 3, 4, 5]
for num in numbers:
print(f"Number: {num}")
Output:
javascriptCopy codeNumber: 1
Number: 2
Number: 3
Number: 4
Number: 5
In this example, the loop runs through each element of the numbers
list and processes it.
Using the range()
Function in a For Loop
Python provides the range()
function to generate a sequence of numbers. This is often used with the for
loop when you need a defined set of iterations.
Example:
pythonCopy codefor i in range(1, 6):
print(f"Square of {i} is {i**2}")
2. While Loop: Repeating Based on a Condition
The while
loop repeatedly executes a block of code as long as the specified condition evaluates to True
. Unlike the for
loop, the number of iterations in a while
loop is not fixed and often depends on dynamic runtime conditions.
Syntax:
pythonCopy codewhile condition:
# Execute this block of code
Use Case:
- When the termination condition is unclear at the start.
- Useful for tasks dependent on user input or events.
Example:
pythonCopy codecounter = 1
while counter <= 3:
print(f"Counter value: {counter}")
counter += 1
Output:
yamlCopy codeCounter value: 1
Counter value: 2
Counter value: 3
Here, the loop keeps running until the condition counter <= 3
is no longer true.
Key Control Flow Statements in Loops
Python provides three powerful keywords to manage and modify the flow of loops. These statements enhance control and flexibility while working with loops:
break
: Terminates the loop prematurely, irrespective of the condition.
Example:pythonCopy codefor num in range(1, 10): if num == 5: break print(num)
Output:Copy code1 2 3 4
continue
: Skips the current iteration and moves to the next iteration of the loop.
Example:pythonCopy codefor num in range(1, 5): if num == 3: continue print(num)
Output:Copy code1 2 4
else
: Used with bothfor
andwhile
loops. Executes once the loop finishes unless terminated by abreak
.
Example:pythonCopy codefor num in range(1, 5): print(num) else: print("Loop complete")
Output:vbnetCopy code1 2 3 4 Loop complete
Summary of Python Loop Types
- For Loop: Best for iterating over sequences with a predetermined number of iterations.
- While Loop: Ideal for indefinite loops where the exit condition depends on external factors or real-time changes.
- Special Keywords: Enhance loop control for specific use cases (e.g., premature termination, skipping, or handling post-loop logic).
By understanding these loop types and their variations, Python developers can effectively automate repetitive tasks, optimize performance, and create dynamic and scalable applications. Whether iterating over a dataset, building an interactive menu, or solving complex problems, loops are an essential tool in Python’s arsenal.