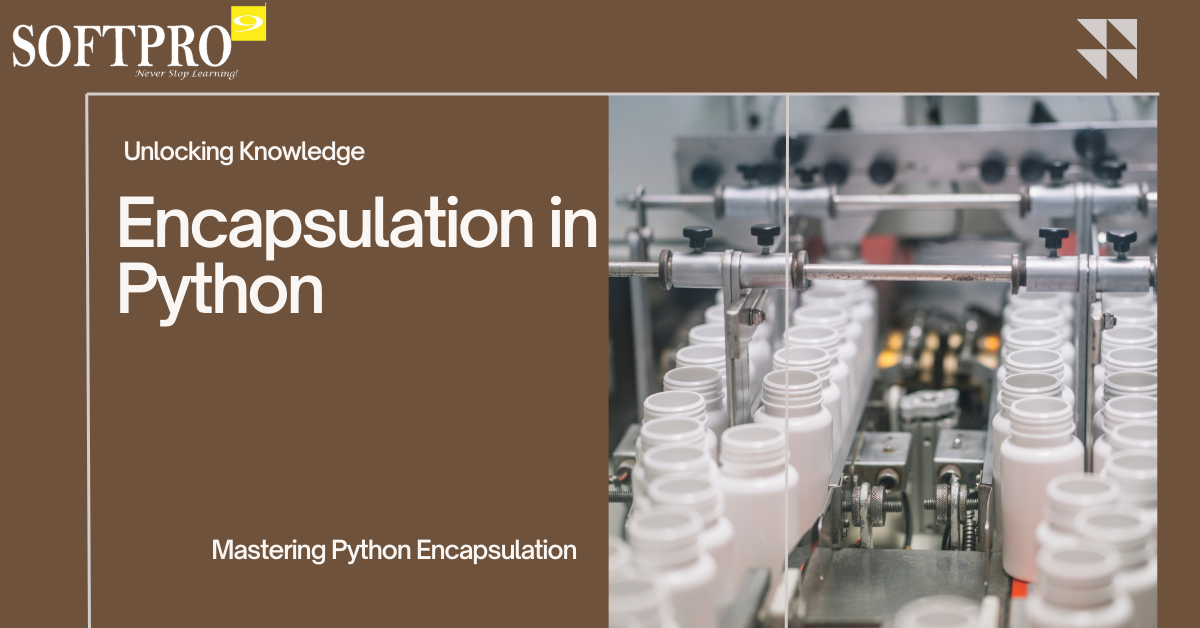
Mastering Encapsulation in Python: Concepts, Benefits, and Coding Examples
#1 Myinstitutes.com is one of the Best Educational Portal and Training Institutes in MYSORE, MANGALORE, and BANGALORE.
Encapsulation is one of the four key principles of Object-Oriented Programming (OOP), alongside inheritance, polymorphism, and abstraction. In Python, encapsulation allows developers to bundle data and related operations within a single unit (typically a class) while controlling how that data is accessed or modified. This principle plays a pivotal role in creating robust and maintainable software systems.
This blog will dive deep into encapsulation in Python, exploring its concepts, benefits, and practical implementation through clear coding examples. By the end, you’ll understand how to use encapsulation to enhance your projects and make your code secure, modular, and easier to manage.
What Is Encapsulation?
Encapsulation refers to the process of wrapping data (attributes) and methods (functions) together in a class while restricting direct access to some of the object’s components. This restriction is achieved by controlling the visibility of data through access specifiers (public, protected, and private). Encapsulation enables secure data management, ensuring only intended interactions with an object’s internal state.
In Python, encapsulation is slightly different compared to strictly-typed languages like Java or C++. Python doesn’t strictly enforce access rules but uses naming conventions to signify access levels. Let’s explore these levels in detail:
Access Specifiers in Python
- Public:
Public members can be accessed from anywhere in the program. By default, all attributes and methods in Python are public unless specified otherwise.Example:pythonCopy codeclass Example: def __init__(self): self.public_attribute = "I am public" obj = Example() print(obj.public_attribute) # Output: I am public
- Protected:
Protected members, denoted with a single underscore_
, are accessible within the class and its subclasses. While not fully private, the single underscore hints that these members are intended for internal use.Example:pythonCopy codeclass Parent: def __init__(self): self._protected_attribute = "I am protected" class Child(Parent): def show_protected(self): print(self._protected_attribute) obj = Child() obj.show_protected() # Output: I am protected
- Private:
Private members, denoted with double underscores__
, are accessible only within the class. This restriction helps secure critical attributes from being accidentally accessed or modified externally.Example:pythonCopy codeclass SecureClass: def __init__(self): self.__private_attribute = "I am private" obj = SecureClass() try: print(obj.__private_attribute) # Raises AttributeError except AttributeError: print("Cannot access private attribute directly!")
Implementing Encapsulation with Getter and Setter Methods
Private attributes often require controlled access, which is achieved using getter and setter methods. These methods enforce data validation and maintain integrity, providing safe ways to interact with the private attributes.
Example: Implementing Encapsulation with Getters and Setters
pythonCopy codeclass BankAccount:
def __init__(self, owner, balance):
self.__owner = owner # Private attribute
self.__balance = balance # Private attribute
# Getter for balance
def get_balance(self):
return self.__balance
# Setter for balance with validation
def set_balance(self, amount):
if amount >= 0:
self.__balance = amount
else:
print("Balance cannot be negative.")
# A method to display account details
def display_account(self):
print(f"Account Holder: {self.__owner}, Balance: ${self.__balance}")
# Using the BankAccount class
account = BankAccount("Alice", 1000)
account.display_account()
# Access and modify the balance using methods
print("Current Balance:", account.get_balance())
account.set_balance(1200) # Valid update
account.display_account()
# Invalid update attempt
account.set_balance(-500)
Benefits of Encapsulation
Encapsulation provides several key benefits, which are vital for maintaining scalable and reliable software systems:
- Security and Data Hiding:
Critical information remains secure and inaccessible to unauthorized users or external code. - Data Integrity:
Encapsulation enforces validation rules before modifying attributes, reducing the risk of invalid data inputs. - Modularity and Code Reusability:
By bundling data and methods, classes become self-contained modules, encouraging code reuse and simplifying debugging. - Flexibility for Future Changes:
Encapsulation allows internal implementation changes without affecting external code that relies on the class interface. - Improved Readability and Maintainability:
It makes the intent of the code clear, ensuring a well-structured, easy-to-maintain design.
Encapsulation Pitfalls to Watch Out For
While encapsulation is an essential programming tool, there are a few challenges to consider:
- Overuse of Private Attributes:
Excessive restriction of access can make the code difficult to debug or extend, especially in collaborative environments. - Confusion Over Naming Conventions:
Python’s use of underscores for protected and private members relies on conventions rather than enforced rules, leading to potential misunderstandings. - Trade-off Between Flexibility and Security:
Striking the right balance between providing flexibility and maintaining security is key.
Conclusion
Encapsulation is a foundational principle for writing clean, secure, and modular Python code. By wrapping data and methods within a class and controlling access, you can build applications that are resilient and easier to manage. Understanding and implementing encapsulation with the help of concepts like access specifiers, getter/setter methods, and data hiding lays the groundwork for mastering Object-Oriented Programming in Python.
Whether you are designing a small utility script or a large-scale software application, embracing encapsulation will elevate your coding practices and help you create systems that stand the test of time.